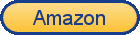
How do you sleep in Javascript? How do you insert a delay of a few seconds when a Javascript function has been run? What is the correct way to pause for a few seconds in Javascript or jQuery?
Below is what you want.
4 | doSomethingHereAfterThreeSeconds(); |
Let's look at the solution below.
Do NOT use the solution where you have a while loop and you check the time stamp in each iteration to see if it has passed the desired amount of time. It will heavily drain your system's resources.
Solution
Simply use Javascript's setTimeout() function. It takes two arguments. The first argument is the Javascript function you want to execute. The second argument is the time interval in milliseconds, which must pass before the said function is executed.
setTimeout() is an asynchronous function and will return immediately when you call it.
Below let's look at some sample code:
2 | function doSomethingHereAfterThreeSeconds(){ |
6 | setTimeout( function(){doSomethingHereAfterThreeSeconds();}, 3000 ); |
This code calls doSomethingHereAfterThreeSeconds() three seconds after it executes doSomethingHere().
Alternatively you can call doSomethingHereAfterThreeSeconds() inline as follows:
Next we will look at some more advanced materials related to this topic.
Question
What if I'd like to call doSomethingHereAfterThreeSeconds() every 3 seconds until some task has been done?
Answer
I am glad you ask that. Please read
How To Guarantee that Facebook SDK Is Loaded on Your Website? for a detailed example of how to call a Javascript function at some time intervals until some task has been done.
Questions? Let me know!